This is the final coding project that was created as part of the Advanced Topics in Programming course at the Ben-Gurion University of the Negev.
​
The course combines theoretical and practical aspects of software development for the creation of windows-based applications (AKA form-based applications) which are also integrated with databases. During the course, theoretical subjects are reviewed such as classical User Interface Design Patterns (e.g., the Observer pattern), and also practical issues such as an overview of the WindowsForms class library. The main topics that are covered include Eventbased programming, using User controls to create friendly user interfaces, creation of custom user controls, Visual inheritance, and MultiDocument Interfaces (MDI). In addition, advanced topics such as using of Webserivces, Deployment issues and Versioning will also be introduced.

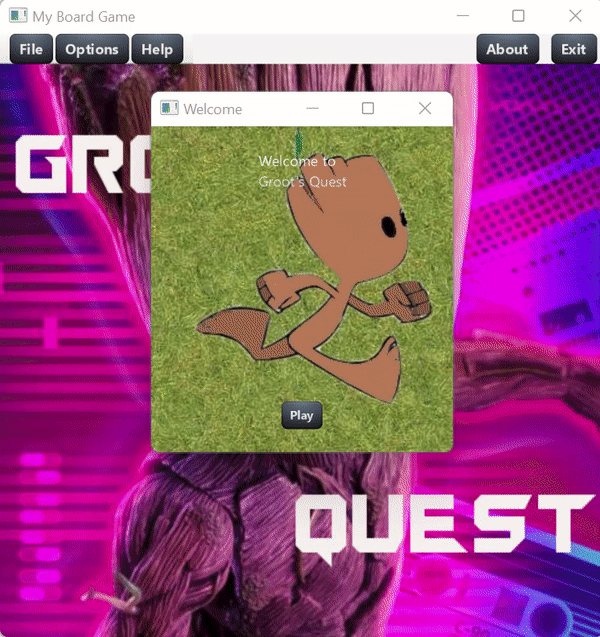
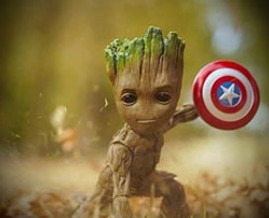
The implemented appplication generates a maze according to a user’s settings. The user can then try to solve it theirselves or ask the program to solve it for them. The project is written from scratch in java 8 using IntelliJ IDEA. We implemented multiple algorithms for maze generation and maze solving, along with client-server architecture using the SOLID software design concepts.
First Phase
Generation of the Maze - link
The maze is generated using a randomized variation of the Prim algorithm.
For the computation of the solution of the maze the following algorithms were implemented to solve it:
-
Breadth First Search
-
Depth First Search
-
Best First Search
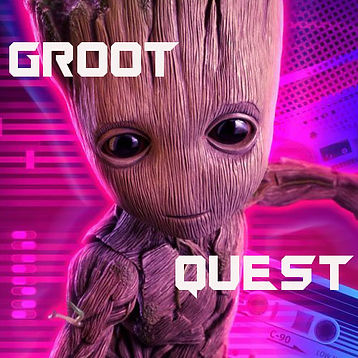
Maze Solution
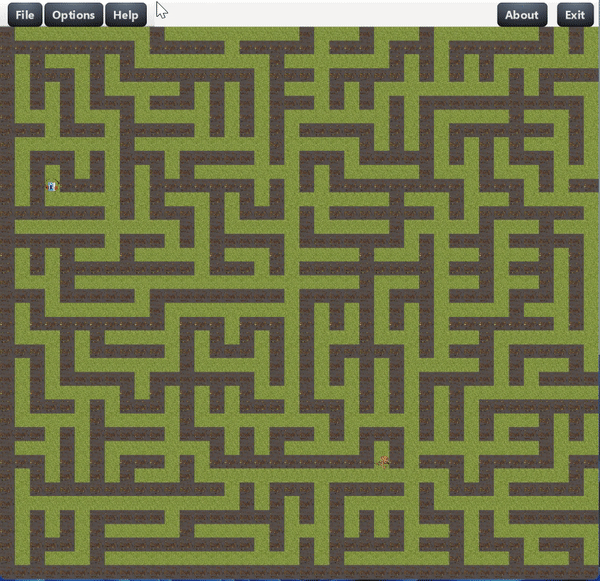
Second Phase
Threads and Streams - link
The project includes a server with two seperate functions:
-
Generate a maze according to the user’s settings - the client sends all the relevant parameters, the server generates the maze and sends it back to the client as an object.
-
Solving the maze
To reduce the communication overheads, we used compression using the Decorator design pattern. The server supportes caching; if the server has to solve a maze which has already been solved in the past, it retrieves the solution from a file and returns the answer to the client without solving it again. The client-server architecture includes a thread-pool mechanism.
The server can support multiple clients in parralel.
To set the optimal settings (number of threads, best algorithm to solve the maze, etc..) we defined a static class called Configurations.
Third Phase
Desktop application using MVVM architecture, GUI - link
-
The Model layer is responsible for the business logic (The server side).
-
The ViewModel layer is responsible for the presentation logic and to mediate between the two other layers.
-
The View layer is responsible for the FXML and the UI logic (The client side).
Using the GUI the user can create a new maze, save the current state of the game, and load an existing game. We also used Maven with Log4j2 library;indications are written to the log file such as valid input, calling to the server, details about the solution, etc..
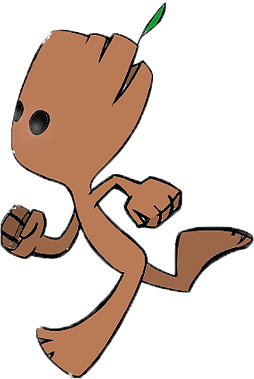